User comments (analysis.comments)
- analysis.comments: malcat.UserComments
The
analysis.comments
object is amalcat.UserComments
instance that gives you access to all the comments added by the user.
Comments are simple text strings added by the user at a given address. They can be viewed in the Hexadecimal view, Structure/text view or Disassembly view (if you have them activated in the Highlighting). They are saved within a .malcat
project. In addition to the UI, comments can also be parsed and edited programmatically as we will see in this chapter.
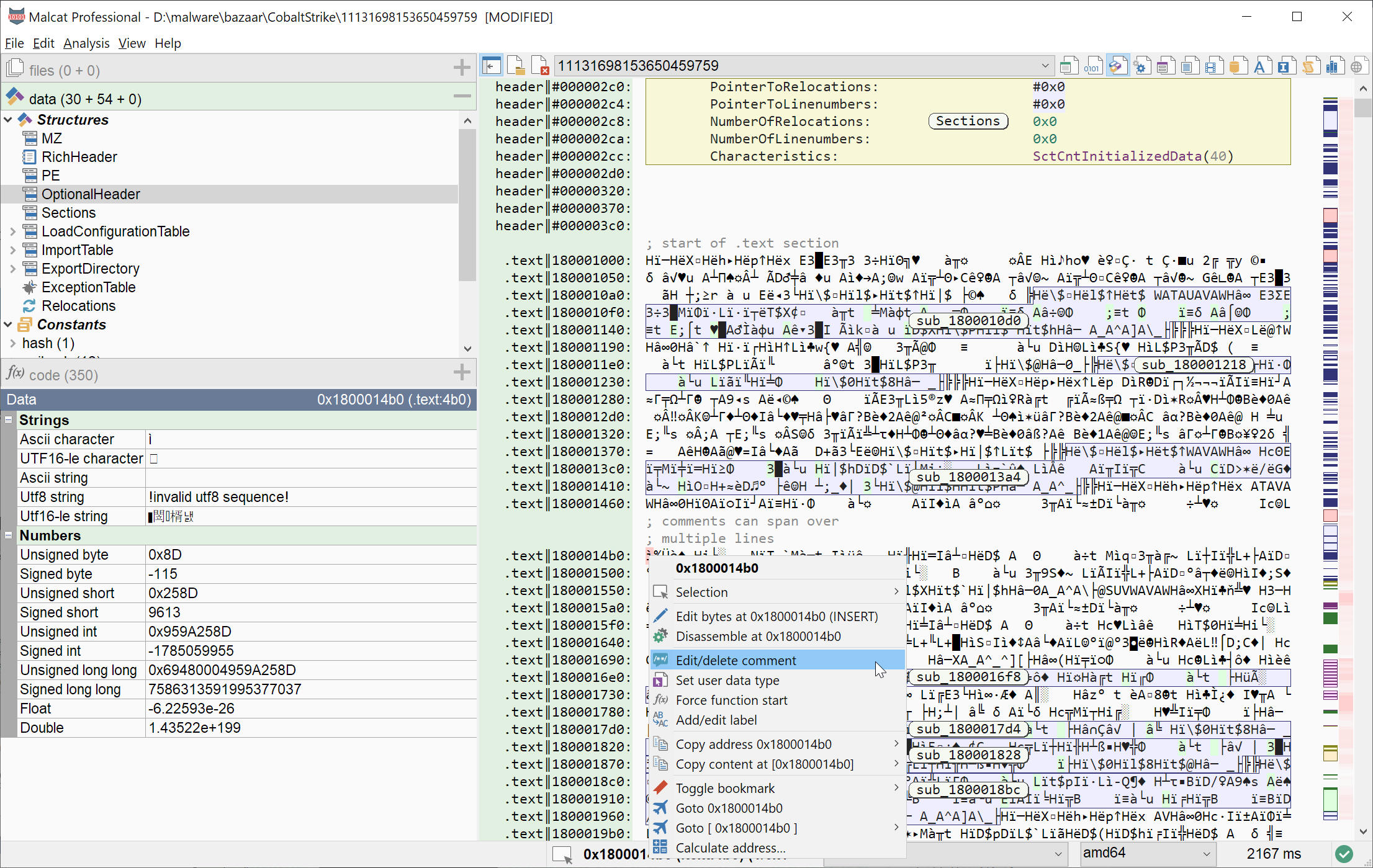
Adding user comments via the UI
User comment object
A user comment is simply an arbitrary text string associated to a given address in Malcat.
- class malcat.UserComment
- __init__(ea, text)
Create a new comment instance, for use with the
UserComments.add()
functionaddress = analysis.v2a(0x401000) c = malcat.UserComment(address, "a comment text") if not analysis.comments.add(c): raise ValueError(f"Could not add comment {c} at {analysis.ppa(address)}")
- param int ea:
the address of the comment
- param str text:
the text of the comment
- address: int (effective address)
the address of the comment
- text: str
the content of the comment. May contains newlines.
Note about editing comments
Undo/redo
Each comment you add gets saved in the list of undoable operations, which can be somewhat expensive if you plan to add thousands of comments. In general, if you plan to do a lot of modifications (comments or other types of edits like file writes for instance), you can chose to deactivate undos:
analysis.history.record(False)
# ... add your comments, they won't appear in the undo list
for i in range(malcat.map.end):
malcat.comments[i] = "..."
# ... reactivate undo/redo
analysis.history.record(True)
Note
Edits which are not recorded to the undo list are invisible to Malcat. It means Malcat will not see that the file has been modified and the UI won’t offer you to save your modifications on quit.
If you stil want to record your edits in the undo/redo but you would like to group them in just one operation, you can do it too:
with analysis.history.group()
# ... add your comments
for i in range(malcat.map.end):
malcat.comments[i] = "..."
analysis.history.undo() # <-- will undo all comments in one go