Anomalies (analysis.anomalies)
- analysis.anomalies: malcat.Anomalies
The
analysis.anomalies
object is amalcat.Anomalies
instance that gives you access to all anomalies found by the Anomaly scanner.
Note that in addition to this documentation, you can find usage examples in the sample script which is loaded when you hit F8.
What are anomalies?
Malcat features a powerful anomaly scanner which inspects the finished analysis object in order to highlight anything suspicious in the file. It is present in all paid versions of Malcat.
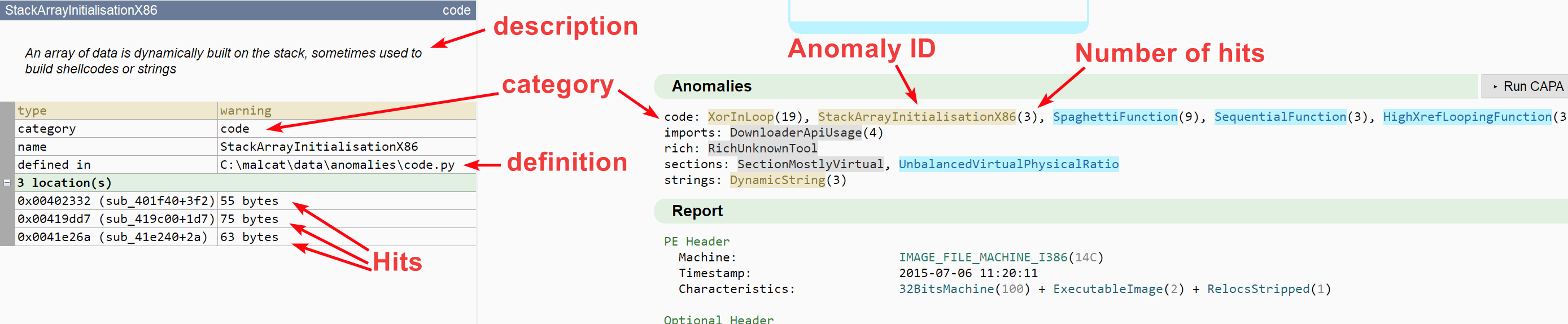
Displaying anomalies found in files
Anomalies are small heuristics computed by several python functions located in data/anomalies
which make use of Malcat’s python Analysis object (analysis). The result is available to the user through the analysis.anomalies
object.
Accessing / enumerating anomalies
- class malcat.Anomalies
This class contains all the anomalies identified by the Anomaly scanner. Note that all addresses used in this class are effective addresses. See Addressing in Malcat for more details.
- __iter__()
Iterate over the file’s identified / matching anomalies
for a in analysis.anomalies: print(f"Anomaly {a.label} [{a.category}] found, locations:") for addr, size in a.locations: print(f" * #{analysis.map.to_phys(addr)} ({size} bytes)")
- Return type:
iterator over
Anomaly
instances
- __getitem__(name)
Returns the first
Anomaly
named name, or None if the anomaly did not match / does not exist.dbg_path_anomaly = analysis.anomalies["EmptyDebugPath"] # equivalent dbg_path_anomaly = analysis.anomalies.EmptyDebugPath
- Parameters:
name (str) – name of the anomaly you are looking for
- Return type:
Anomaly
instance orNone
- __getattr__(name)
Returns the first
Anomaly
named name, or None if the anomaly did not match / does not exist.dbg_path_anomaly = analysis.anomalies.EmptyDebugPath # equivalent dbg_path_anomaly = analysis.anomalies["EmptyDebugPath"]
- Parameters:
name (str) – name of the anomaly you are looking for
- Return type:
Anomaly
instance orNone
- __contains__(name)
Returns True iff an anomaly named name exists and matches at least once in the current file
if "NoValidCertificate" in analysis.anomalies: print("the certificate entry looks corrupted")
- Parameters:
name (str) – name of the anomaly you are looking for
- Return type:
bool
The anomaly object
- class malcat.Anomaly
This class represents an anomaly that matched against the current file.
- name: str
name of the anomaly, this is the python class name of the corresponding scanning function in
data/anomalies
.
- comment: str
long textual description fo what the anomaly tries to detect
- category: str
category of the anomaly, informal. Can be “resource”, “entropy”, “code”, etc.
- level: malcat.Anomaly.Level
dangerosity of the anomaly. Can be one of:
- locations: List[int, int]
all the file intervals where the anomaly was detected. This is a list of (effective address, size). Note that a few anomalies are detected at the file level and have no particular location per se.
The level / dangerosity of an anomaly can be:
- class malcat.Anomaly.Level
- TRACE
this is not really an anomaly, more like a noteworthy property of the file
- ODD
this anomaly may appear in both clean an malicious files, but not that often
- WARNING
this anomaly is seen in a lot of malicious files, and rarely in clean files. Please inspect.
- ERROR
this anomaly is seen almost exclusively in malicious files.