User highlighted regions (analysis.highlights)
- analysis.highlights: malcat.UserHighlights
The
analysis.highlights
object is amalcat.UserHighlights
instance that gives you access to all the regions highlighted by the user.
Highlighted regions are simple text are regions which were given a title, a note text and a category by the user. They can be viewed in the Hexadecimal view or the Structure/text view (see Highlight/annotate a region). They are saved within a .malcat
project. In addition to the UI, highlighted regions can also be parsed and edited programmatically as we will see in this chapter.
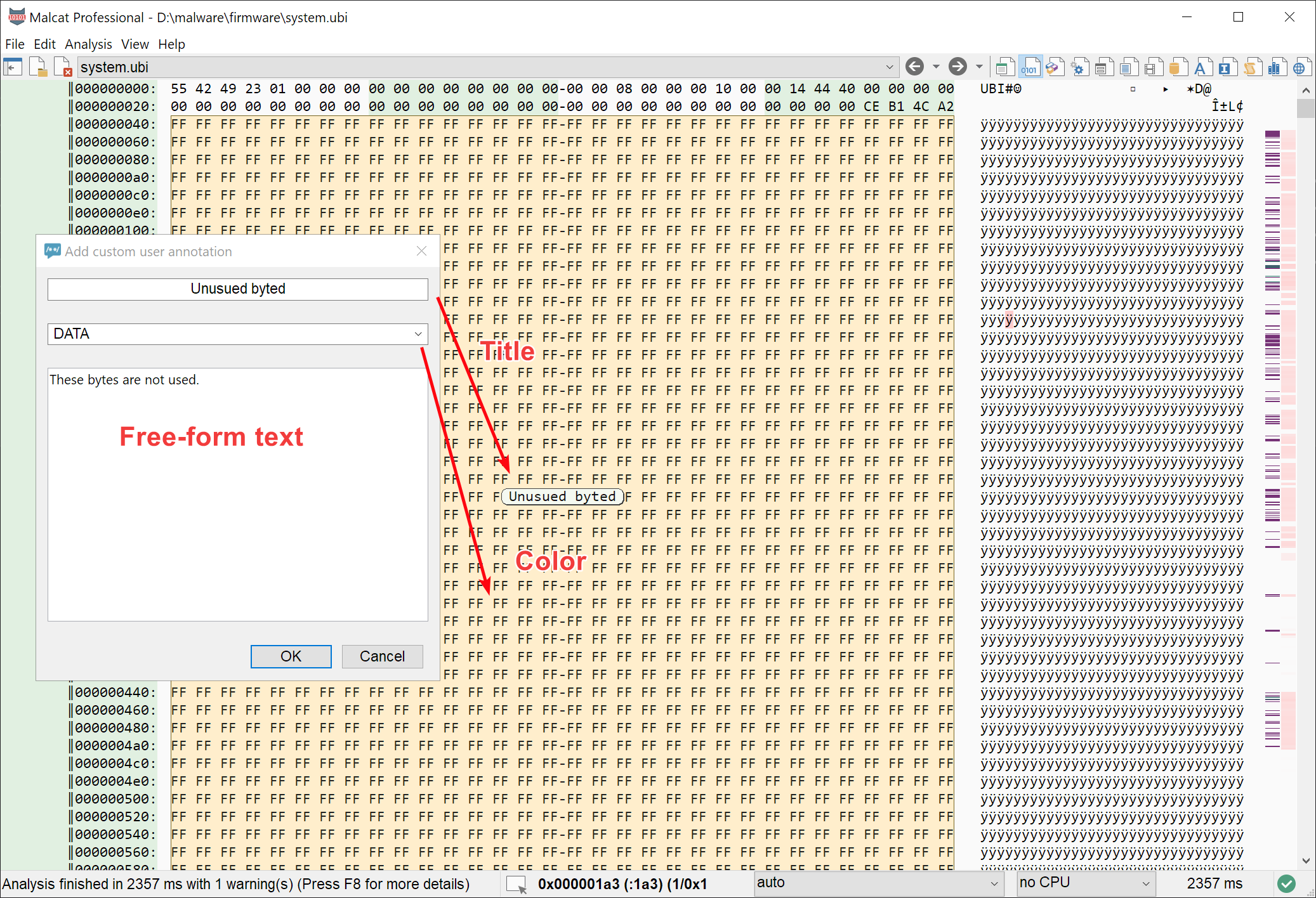
Highlighting user regions using the UI
User highlighted region object
A user highlighted region is a file interval which has been given a title, a comment and a category by the user:
- class malcat.UserHighlight
- __init__(ea, size, title, text='', category=malcat.Category.DEBUG)
Create a new highlighted region instance, for use with the
UserHighlights.add()
functionaddress_start = analysis.v2a(0x401000) address_end = analysis.v2a(0x402000) c = malcat.UserHighlight(address_start, address_end - address_start, "title", "a comment text", malcat.Category.DEBUG) if not analysis.highlights.add(c): raise ValueError(f"Could not highlight user region [{analysis.ppa(address_start)}-{analysis.ppa(address_end)}[")
- param int ea:
the start address of the region to highlight
- param int size:
the size of the region
- param str title:
the title of the region
- param str text:
the comment for the region
- param malcat.Category category:
the category of the region
- address: int (effective address)
the address of the highlighted region
- size: int
the size of the highlighted region
- title: str
the title of the highlighted region
- content: str
the content of the comment. May contains newlines.
The category of a highlighted region dictates its color in the UI and wether it will be displayed or not, depending on Highlighting.
Note about editing user highlights
Undo/redo
Each highlighted region you add gets saved in the list of undoable operations, which can be somewhat expensive if you plan to add thousands of highlighted regions. In general, if you plan to do a lot of modifications (highlighted regions or other types of edits like file writes for instance), you can chose to deactivate undos:
analysis.history.record(False)
# ... add your highlighted regions, they won't appear in the undo list
for i in range(malcat.map.end):
malcat.highlights[i:i+1] = "..."
# ... reactivate undo/redo
analysis.history.record(True)
Note
Edits which are not recorded to the undo list are invisible to Malcat. It means Malcat will not see that the file has been modified and the UI won’t offer you to save your modifications on quit.
If you stil want to record your edits in the undo/redo but you would like to group them in just one operation, you can do it too:
with analysis.history.group()
# ... add your highlighted regions
for i in range(malcat.map.end):
malcat.highlights[i:i+1] = "..."
analysis.history.undo() # <-- will undo all highlighted regions in one go